#28 Python Programming – Conditional Statements and Dictionary
Python Programming – Conditional statements and Dictionary

Python conditional statements
Page Contents
Python Conditional Statements
if statement
- The if statement contains a logical expression using which data is compared and a decision is made based on the result of the comparison
if( condition-expression ):
Syntax :
if( condition-expression ):
Operation Block
- If the Boolean expression evaluates to True, then the block of statement(s) inside the if statement is executed.
- If Boolean expression evaluates to False, then the first set of code after the end of the if statement(s) is executed.
- Note: The indentation is important in python. After conditional statement, on the next line followed by indent, write down true block operation
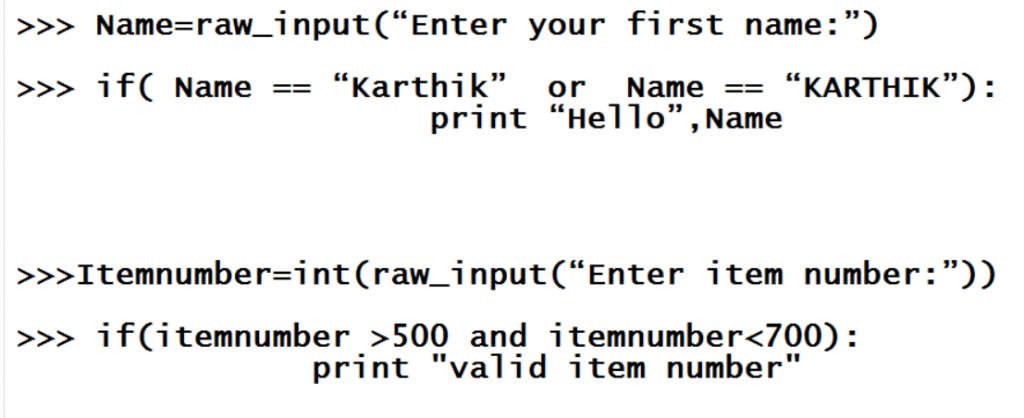
if..else style
- An else statement can be combined with an if statement
- If the condition is not True , the control will go to the else block part
Syntax :
if (condition):
True Block operation
else:
False Block operation
- Note: After the else statement, the next line followed by the indentation is the False block operation
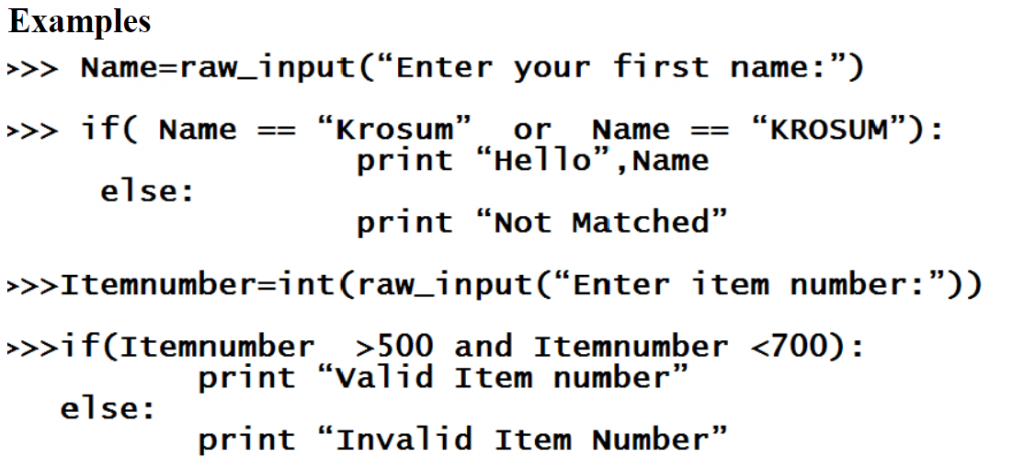
if..elif..elif..else style
- Based on the outcome, if we need to determine which action to take place and which statements to execute, then the choice will be Multi conditional statements
Syntax:-
if ( condition1) :
True block -1
elif ( condition2):
True Block-2
elif (condition3):
True Block-3
…
elif (conditionN):
True Block-N
else:
False Block
- Python will test the condition1 expression, if condition1 is True, the control will go to Trueblock -1, executes the python code then exits from the block
- If condition1 fails, then the control will test the condition2 block
- If condition2 is True, execute True Block-2 operation, then exit from control statement so on
- Only if all the conditions are failed control will go to else block
Example :
if ( “AB” == “AB”) :
print (“Im True Block1”)
elif (100 >50):
print (“Im True Block2”)
elif (“SAB” != “sab”) :
print (“Im True Block3”)
else :
print (“False Statement”)
- In the above example, all the given conditions are True
- As per the flow, the Python will test the 1st condition ( “AB” == “AB”)
- Here condition1 is True, so the control will go to True block
- In case if 1st condition is False, the control will go to next condition
Result : “Im True Block1”
Looping in Python
- A looping statement allows us to execute the code blocks more than one time
- Python looping statements are executed in two ways
- List style – Execution of the block depends on the number of elements in the list
- Conditional style – – Execution of the block depends on the conditional statement
Python for loop
Syntax
for variable in list-of-elements:
Operation block
# Note:
Just like conditional statements, looping statements also needs proper indentation.
for, in are keywords.
- If a list-of-elements in turn contains a list or a tuple or an unnamed expression list, the loop evaluates them first
- And then, the first item in the list is assigned to the iterating variable
- Next, the statements block is executed.
- Further, each item in the list is assigned to iterating variable, and the statement(s) block is executed until the entire list is finished
Example 1
for var in "host01","host02","host03" :
print "Host name:",var # Block will run 3 times
Result :-
Host name:host01
Host name:host02
Host name:host03
Example 2
IPs=['10.20.30.40','10.20.30.50','10.20.30.60','10.20.30.66','10.20.40.60']
for var in IPs:
print "System IP:",var
print "-------------------"
Result:-
System IP: 10.20.30.40
———————————–
System IP:10.20.30.50
———————————–
System IP:10.20.30.60
———————————–
System IP:10.20.30.66
———————————–
System IP:10.20.40.60
- The above looping statement will run 5 times
- List variable IPs is holding 5 elements so block will run 5 times
Example 3
# Display the CPU Load Balance for 5 times
import os
for v in 1,2,3,4,5:
os.system("uptime")
Example 4
import os
for v in [1,2,3,4,5]: <======= List contains 5 elements
os.system("uptime")
Example 5
import os
for v in (1,2,3,4,5): <======= tuple contains 5 elements
os.system("uptime")
Example 6
import os
for v in ('data1','data2','data3','data4','data5'): <======= tuple contains 5 elements
os.system("uptime")
- All the above examples have same result.
- It displays the current CPU load balance result 5 times
range()
By using the range() method, we can iterate over the looping operations, The range function takes number (any integer),say n as argument and returns a list of values start from 0 to n-1
Example:
range(5) ==> [0,1,2,3,4]
range(n,m) ==> start from 'n' value to 'm-1' value
range(1,5) ==> [1,2,3,4]
range(n,m,count) ==> start from 'n' value to 'm-1' for every count occurrence
range(1,7) ==> start from 1 to 6 ==> [ 1,2,3,4,5,6 ] ==> for every occurrence single increment
range(1,7,3) ==> every 3 increment ==> [1,4]
How to use range() in for loop statements ?
Example:
for v in range(5) : # [0,1,2,3,4] No.of elements in the list is 5 ,so block will run 5 times
print "Hello..",v
Result:-
Hello..0
Hello..1
Hello..2
Hello..3
Hello..4
for v in range(1,10,3):
print "value of v:",v # Block will run 3 times
Result :-
value of v:1
value of v:4
value of v:7
Using else Statement with Loops
- Python supports the usage of an else statement with a loop statement
- In case the else statement is used with a for loop, the else statement is executed when the loop has finished iterating the list
- Generally else statements are optional in looping statements and are usually used to write a footer message
- else block can be used with both for and while loops
Example:-
for v in range(5):
print "Hello-->",v
else:
print "Thank you"
Result:-
Hello–>0
Hello–>1
Hello–>2
Hello–>3
Hello–>4
Thank you
Python While loop
A while loop in Python continuously executes a statement as long as the given condition is true.
syntax
While (condition ) :
Operation –block
- The Loop will test the condition. If condition is True, the operation block is executed and again the condition is tested and if condition is True again, it repeat the operation
- This goes on until the condition fails where the loop terminates
- Here the operation block execution is determined only by the condition and not by the list, tuple or list of values
Example:-
count=0
while(count<5):
print "Count value :",count
count=count+1
Result :
Count value : 0
Count value : 1
Count value : 2
Count value : 3
Count value : 4
While with else statement
Example :-
count=0
while(count<5):
print "Count value :",count
count=count+1
else :
print "Thank you"
Result
Count value : 0
Count value : 1
Count value : 2
Count value : 3
Count value : 4
Thank you
Looping statements
1) break – Terminate from loops
- The most common use of break is when some external condition is triggered requiring a hasty exit from a loop
- The break statement can be used in both while and for loops
Example
while True:
Fname=raw_input( “Enter a first name:”):
if( Fname == “Ram” ):
print “Matched”
break # Exit from while loop
- The above loop is an un-conditional ( always true ) infinite loop
- Once the condition is matched, block will get terminate
2) Continue-continues on the next iteration of the loop
- It returns the control to the beginning of the while loop
- The continue statement rejects all the remaining statements in the current iteration of the loop and moves the control back to the top of the loop
- The continue statement can be used in both while and for loops
Example :
for var in [‘Mon’,’Tue’,’Wed’,’Thu’,’Fri’]:
if(var == “Wed”):
continue # Skip the wed, continue to the next statement
else:
print var
Result:
Mon
Tue
Thu
Fri
3) Pass statement
- The pass statement is a null operation; nothing happens when it executes
- The pass is also useful in places where your code will eventually go, but has not been written yet (e.g., in stubs for example) ?
Example:–
for letter in 'Python':
if letter == 'h':
pass
print 'This is pass block'
print 'Current Letter :', letter
print "Good bye!"
Result:-
Current Letter : P
Current Letter : y
Current Letter : t
This is pass block
Current Letter : h
Current Letter : o
Current Letter : n
Dictionary
- Python dictionary is an unordered collection of data items
- Like list python dictionary is also modifiable
- A dictionary contains collection of items, which is of (key,value) pair
Syntax :-
Dictionaryname={‘Key’ : ‘Value’ }
- Keys are unique string whereas value can duplicate
- Each key is separated from its value by a colon (:), the items are separated by commas, and the whole thing is enclosed in curly braces
- The values of a dictionary can be of any type, but the keys must be of an immutable data type such as strings, numbers, or tuples
How to create a dictionary?
- Creating a dictionary is as simple as placing items inside curly braces { } separated by comma
Syntax :-
Dictionaryname={‘Key1’ : ‘Value1’, ‘Key2’: ‘Value2’…….’Key N’: ‘Value N’ }
Examples:-
Hosts={‘ip’ : ’10.20.30.40’, ‘file’:’/etc/hosts’ , ‘port’ : 22 }
Hosts is the name of the dictionary
ip is a key & its value is an IP address (10.20.30.40 )
file is a key & its value is /etc/hosts
port is a key & its value is 22
Generally, dictionaries have a key and the corresponding value expressed as a pair, key: value
How to access the elements from a dictionary?
- Similar to list, in order to get the data from a dictionary, we use index [ ] notation
Using index [ ] :
dict_name[‘key’]
- Keys can also be used with the get() method to access the elements from a dictionary.
Using get() :
dict_name.get(‘key’)
Examples:-
emp={‘name’ : ‘Mr.Kris’ , ‘ID’ : ‘E123’, ‘dept’:’sales’}
print “Emp name is:”, emp[‘name’]
print emp[‘name’],” Working department is:”,emp.get(‘dept’)
Mr.Kris Working department is: sales
Size of a dictionary
- We use len() function to display the size of a dictionary
emp={‘name’ : ‘Mr.Vishnu’ , ‘ID’ : ‘E123’, ‘dept’:’sales’}
print len(K)
3
- Data inside a dictionary are unordered elements
- In order to get list of keys from dictionary, we use keys() function
Syntax:-
dictionary_name.keys()
keys() returns a collection of values and its return type will be of list type.
K=emp.keys()
# Determine keys() return type
type(K)
(or)
type(emp.keys())
Dictionary Manipulation
Dictionary are mutable, which means that we can add new data (key:value) into an existing dictionary or we can update/modify the value of an existing dictionary using an assignment operator
Adding new data to a dictionary
Whenever adding a new data to an existing dictionary, we should define a new unique key and a value
Syntax :-
dictionary_name[‘Key’]=”Value”
Examples:-
>>>emp={‘name’ : ‘Mr.Vishnu’ , ‘ID’ : ‘E123’, ‘dept’:’sales’}
>>>print len(emp.keys())
3
>>>emp[‘phone’]=”+91 9901233456”
>>>emp[‘place’]=”Bangalore”
>>>print len(emp.keys())
5
Modifing an existing data in a dictionary
Syntax:-
dictionary_name[‘Key’]=”Updated Value”
Example:-
>>>emp={‘name’ : ‘Mr.Vishnu’ , ‘ID’ : ‘E123’, ‘dept’:’sales’, ’phone’ : ’+91 9901233456’, ‘place’ : ‘Bangalore’}
>>>print “Before Modification : “, emp[‘dept’]
>>>emp[‘dept’]=”PRODUCTION” # modify existing value
>>>print “After Modification : ”,emp[‘dept’]
Result:-
After Modification : PRODUCTION
Before Modification : sales
Delete a single record from dictionary
Syntax :- del(dictionary_name[‘Key’])
To clear the entire contents of a dictionary
Syntax:-
dictionary_name.clear( )
Example:-
>>>emp={‘name’ : ‘Mr.Vishnu’ , ‘ID’ : ‘E123’, ‘dept’:’sales’}
>>>print “Total no.of records :”,len(emp.keys())
Total no.of records : 3
>>>del(emp[‘dept’] ) # to delete a single record
>>>print “Total no.of records :”,len(emp.keys())
Total no.of records : 2
>>>emp.clear( ) # remove all entries in emp dictionary
>>>print “Total no.of records :”,len(emp.keys())
Total no.of records : 0
To delete an entire dictionary
del(emp)
Dictionary methods and examples
Syntax
dictionary_name.clear() #removes all elements of dictionary
>>>emp={‘name’ : ‘Mr.Vishnu’ , ‘ID’ : ‘E123’, ‘dept’:’sales’}
>>>emp.clear() - Now the size of dictionary is zero
Syntax : dictionary_name.setdefault(key,default=None)
- The method setdefault() is similar to get(), but the difference is that it sets dictionary_name[key]=default if the key does not exist in the dictionary
- Similar to get() method, it returns the value corresponding to the key
- In case if the given key is not available; it returns the provided default value
emp={‘name’:’Vishnu’,’dept’:’sales’}
emp.setdefault(‘name’) # displays name (key) value as Vishnu.
#If passed as invalid key as an argument , it will return as None.
emp.setdefault(‘Name’) -> None
if(emp.setdefault(‘Name’)==None):
print “Invalid Key”
- The above program input key (‘Name’) is an invalid key, so the default value (None) will be returned
- Here the condition is True .So the control goes to True block and the script say ‘Invalid key’
if(emp.setdefault(‘name’) ==None):
print “Invalid key”
else:
print “value of name is :”,emp[‘name’]
In the above code, the given key is a valid key (‘name’). Hence it returns a string(“Vishnu”) and not ‘None’.
value of name is: Vishnu
Syntax
dictionary.update(dict2)
- The method update() adds the dictionary dict2’s key-values pairs in to dict
- This function does not return anything
- But all items of dict2 is added into dict1
Items={‘code’:’P-123’,’cost’:1000.54}
Files={‘fname’:’Invoice.txt’, ‘size’:’100M’}
Items.update(Files) # Files dictionary is added into Items dictionary
>>> Items
{‘code’:’P-123’,’fname’:’Invoice.txt’,’cost’:1000.54,’size’:’100M’}
# Note that dictionary are unordered data type
>>> print “Total no.of records :”,len(Items.keys())
Total no.of records:4
Syntax
dictionary_name.keys()
- This method returns a list of all keys available in a given dictionary
>>>emp={‘name’ : ‘Mr.Vishnu’ , ‘ID’ : ‘E123’, ‘dept’:’sales’}
>>>emp.keys()
[‘name’, ’ID’, ’dept’ ]
- The return type of keys() will be always list type of data
Syntax
dictionary_name.items()
- The method items() returns a list of dictionary’s (key, value) tuple pairs
>>> emp={‘name’ : ‘Mr.Vinu’ , ‘ID’ : ‘E123’, ‘dept’:’sales’}
>>> emp.items()
[('dept', 'sales'), ('name', 'Mr.Vinu'), ('ID', ‘E123')]
Syntax:
dictionary_name.has_key(Key)
- This method has_key() returns True if a given key is available in the dictionary, otherwise it returns a False.
>>> emp={‘name’ : ‘Mr.Vinu’ , ‘ID’ : ‘E123’, ‘dept’:’sales’}
>>> emp.has_key(‘name’)
True
>>> emp.has_key(‘NAME’) # invalid input key
False
- Since dictionary_name.has_key(Key) returns a Boolean values (True / False ), it is mostly used in testing or validation
if(emp.has_key(‘name’)): # input key is valid return will be True
print “Name is Found:”,emp[‘name’]
else:
print “Invalid key”
- Since the condition satisfies, the above code displays ‘Name is Found: Mr.Vinu’
if(emp.has_key(‘NAME’)): # input key is invalid return will be False
print “Valid Key”
else:
print “Invalid Key”
- Here the function returns false and hence it displays ‘Invalid Key’.
Other dictionary methods
pop() method
- The pop() method removes and returns an element from a dictionary having the given key.
syntax :
dictionary.pop(key[, default])
- Similar to deleting a single key del(dict[‘key’])
emp={‘name’ : ‘Mr.Vinu’ , ‘ID’ : ‘E123’, ‘dept’:’sales’}
>>> emp.pop(‘dept’) # same as del (emp[‘dept’])
sales
>>> emp
{‘ID’:’E123’,’name’:’Mr.Vinu’}
pop( ) Parameters
- The pop() method takes two parameters:
- key – key which is to be searched for removal
- default – value which is to be returned when the key is not in the dictionary
- Return value from pop()
- The pop() method returns:
- If key is found – remove/pop the element from the dictionary
- If key is not found – value specified as the second argument (default)
- If key is not found and default argument is not specified – KeyError exception is raised
emp={‘name’ : ‘Mr.Vishnu’ , ‘ID’ : ‘E123’, ‘dept’:’sales’}
>>> emp.pop(‘ID’)
‘E123’
>>>emp.pop(‘post’,’manager’)
‘manager’
>>>emp.pop(‘K1’) # invalid key
KeyError: ‘K1’
popitem()
The popitem() returns and removes an arbitrary element (key, value) pair from the dictionary.
syntax:-
dict.popitem()
The popitem() doesn’t take any parameters
Return Value from popitem()
- returns an arbitrary element (key, value) pair from the dictionary.
- When you run the program, the output will be
>>>emp={‘name’ : ‘Mr.Vishnu’ , ‘ID’ : ‘E123’, ‘dept’:’sales’}
>>>emp.popitem()
(‘ID’,’E123’)
>>>emp
{‘dept’ : ‘sales’, ‘name’ : ‘Mr.Vishnu’ }
>>>emp.popitem()
(‘dept’:’sales’)
>>>emp
{‘name’ : ‘Mr.Vishnu’}
>>>emp.popitem()
(‘name’ : ‘Mr.vishnu’)
>>> emp
{} # empty dictionary
>>> emp.popitems()
KeyError : ‘popitem(): dictionary is empty’
Reference
Continue exploring at Teknonauts.com
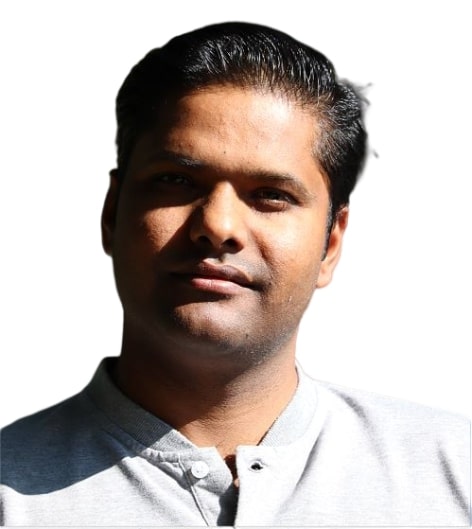
Awadhesh Pratap Dwivedi is an IT industry leader with over 13+ years of experience. He is excellent at providing an easy solution to complex business problems with his tremendous problem-solving skills. Currently, he is working with Oracle as a Principal Solution Engineer.