#25 Python Programming – Setting up system P01
Basics of Python Programming and Setting up the environment

If you are just starting your journey as a Programmer, then I highly recommend you to start with Python. Here are some reason-
Page Contents
Why you should Learn Python
- First of all, Python is an easy-to-learn, interpreted programming language.
- Furthermore, it has efficient high-level data structures, which allow you to write complex operations in fewer statements than in C, C++, or Java.
- Object-oriented programming is a lot easier than in languages like Java.
- Python has become one of the most popular programming languages among developers and programmers because of its Interactive and Extendable features. They praise it for its clean syntax and code readability.
- Python is a general-purpose, high-level programming language.
- Python is both object-oriented and imperative and it can be even used in a functional style as well.
- Python programs are portable, i.e. they can be ported to other operating systems like Windows, Linux, Unix, and Mac OS X, and they can be run on Java and .NET virtual machines.
- Emerging technologies like AI, ML, and Data Science are mostly developed using Python
A Brief History of Python
- Invented in the Netherlands, the early 90s by Guido van Rossum
- Named after Monty Python
- Open-sourced from the beginning
- Considered a scripting language, but is much more
- Scalable, object-oriented and functional from the beginning
- Used by Google from the beginning
- Increasingly popular
Python’s Benevolent Dictator For Life
“Python is an experiment in how much freedom program-mers need. Too much freedom and nobody can read another’s code; too little and expressive-ness is endangered.” – Guido van Rossum
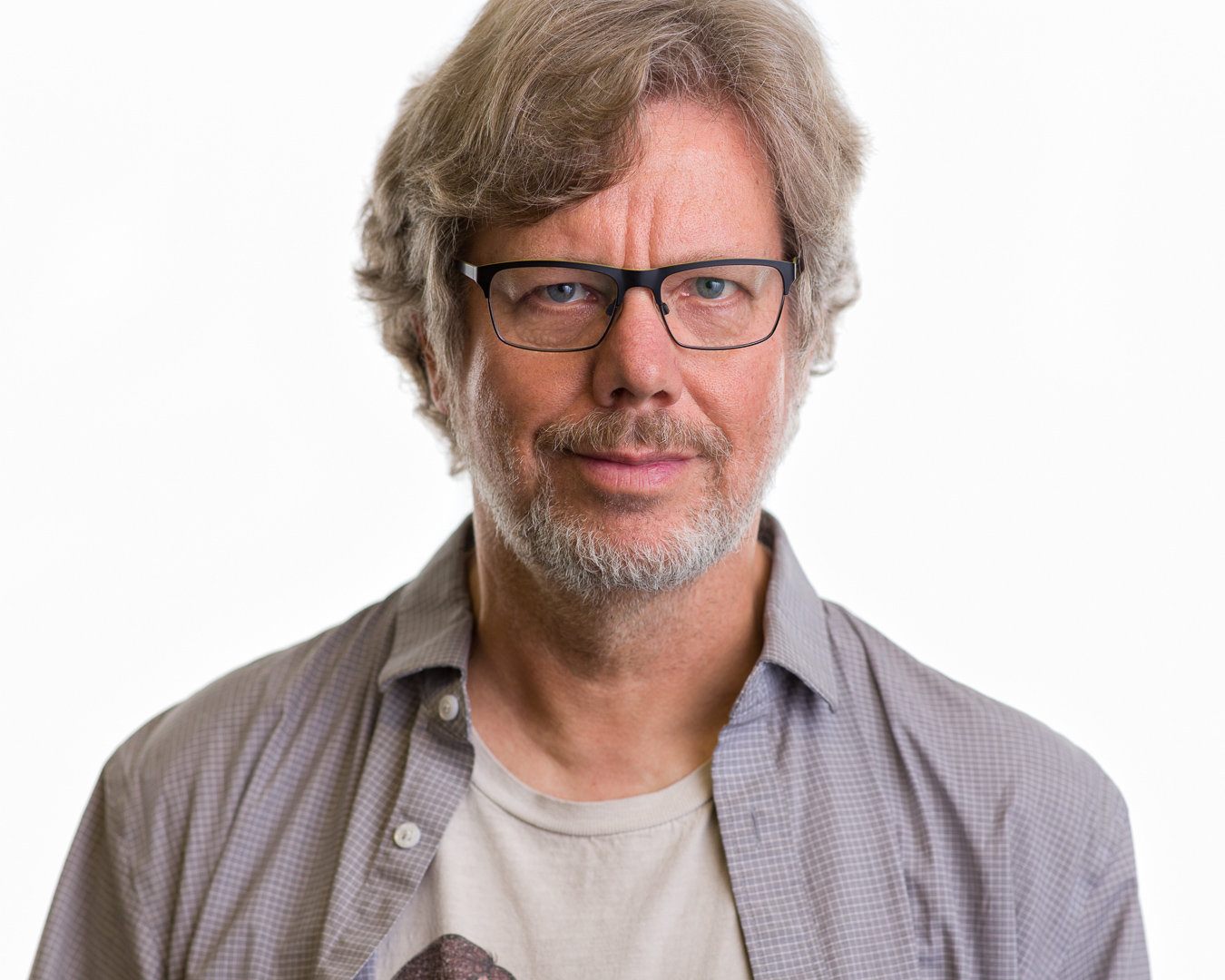
Advantages of Python
- It’s surprisingly easy to embed Python, or better the Python interpreter into C programs.
- The Python Standard Library contains an enormous number of useful modules and is part of every standard python installation.
- We can write python program in object-oriented and procedure style
- Python is a dynamic type language
- Provides interface to all major databases
- Automatic Garbage collection
- We can execute python in different ways
Get Started with Python
Download and install python from – https://www.python.org/
Python IDEs
Komodo | Windows/Linux/Mac OS X | Multi-language IDE with support for Python 2.x and Python 3. Available as Komodo IDE (commercial). |
PyDev | Eclipse | Free, open-source plugin for Eclipse — Allows Python, Jython, and IronPython editing, code-completion, debugger, refactoring, quick navigation, templates, code analysis, unittest integration, Django integration, etc. |
Other popularly used IDEs are Notepad++, Spyder, Jupyter Notebook, Pycharm and Atom
Using the Python Interpreter
- With the Python interactive interpreter, it is easy to check Python commands
- The Python interpreter can be invoked by typing the command “python” without any parameter followed by the “return” key at the shell prompt.
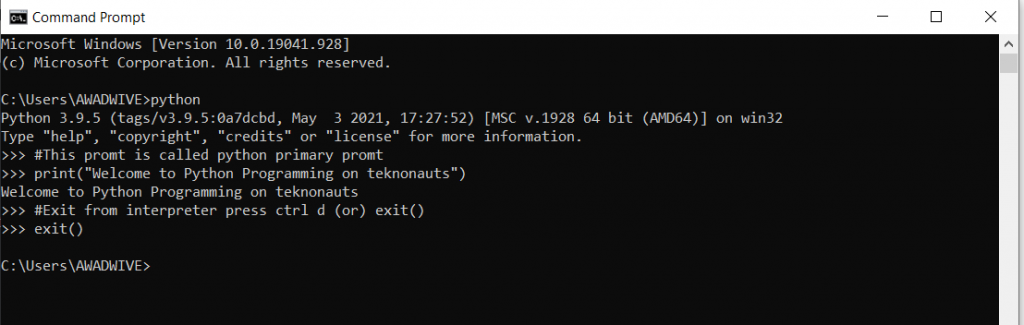
Python Basic
We use the Python interpreter as a simple calculator by typing an arithmetic expression
<p>root@krosumlabs~]# python {Enter-key}</p>
>>> 12+2
37
>>> 2.40+0.25</p>
2.65
>>> “AB” + “SAB” # string concatenation
ABSAB
>>> 5*3 # multiplication
15
>>>”AB”*3 # string repetition
ABABAB
>>> 12.0 / 7 # floating point division
1.7142857142857142
>>>”AB” + 12 #Error- mixed types
- Python follows the usual order of operation in the expression
- The standard order of operations is expressed in the following enumeration
- Exponents and roots
- Multiplication and division
- Addition and subtraction
This means that we don’t need parenthesis in the expression :3 + (2 * 4)
>>> 3 + 2 * 4
11
- Python provides numerous built-in functions (BIFs). Of which help () provides us with the help document on the related object.
>>>help (3)
# Help on int object……
>>>help (int)
# Help on int object……
>>>help (str)
# Help on string object……
- Some other commonly used BIFs are type (), max () and dir ().
>>>type (12)
>>>type(‘12’) #data within quotes are considered to be string .
>>>max(12, 3.145678, 0.2344)
12
>>>dir (10) #returns a list of data & functions associated with the object passed, here an integer (10).
- Usage of the semicolon as a statement terminator is optional in python. However, it can be used to concatenate multiple statements in a single line
>>> 12+3; ‘Learning Well’; 33+7.222
15
‘Learning Well’
40.222
Python Comment Lines
Single Line Comments
- Typically, you just use the # (pound) sign to comment out everything that follows it on that line.
>>> print("Not a comment")
Not a comment
>>> # print("Am a comment") # one line comment
>>> import os # loaded os module
>>> os.system("uptime") # using system function display cpu load balance
Multiple Line Comments
- Multiple line comments are slightly different
- Simply use triple quotes before and after the part that we want to comment
- It can be either triple single quotes (”’…….”’) or triple double quotes (“““…..”””)
''' print("We are in a comment line")
print ("We are still in a comment line")
'''
print("We are out of the comment")
Execute a Python script
- Now Let us save our program in a source file
- To save and edit programs in a file, we need an editor
- There are lots of editors, but you should choose one, which supports syntax highlighting and indentation
- Under Linux, you can use vi, vim, emacs, geany, gedit and umpteen others. So, after you have chosen your editor, you can input your mini script and save it as filename.py
- The suffix .py is not really necessary under Linux but it’s good style to use it
- But it is essential, if you want to write modules
root@krosumlabs~]# vi ab.py
print “Hello this 1st python code”
print “There are many data structures used in python”
# this line single line comment
# python won’t read this line
‘‘‘
This is multiline comment
’’’
print “End of the script”
( Save the program, exit from editor ESC :wq )
root@krosumlabs~]# python ab.py # execute python file
Hello this 1st python code
There are many data structures used in python
End of the script
Structuring with Indentation
- Python uses a different principle
- Programs get structured through indentation; this means that code blocks are defined by their indentation
- All statements with the same distance to the right belong to the same block of code,i.e. the statements within a block line up vertically
- The block ends at a line less indented or the end of the file
- If a block has to be more deeply nested, it is simply indented further to the right
Reference
Continue exploring at Teknonauts.com
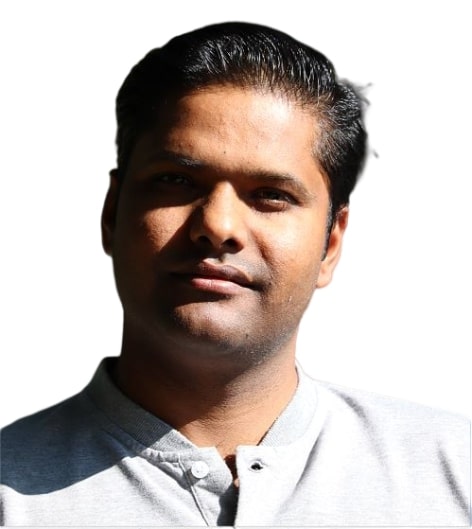
Awadhesh Pratap Dwivedi is an IT industry leader with over 13+ years of experience. He is excellent at providing an easy solution to complex business problems with his tremendous problem-solving skills. Currently, he is working with Oracle as a Principal Solution Engineer.